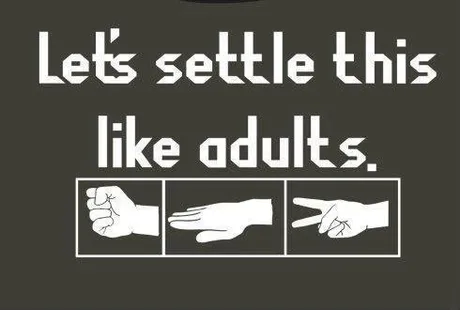
In my spare time for the last week I've been learning JavaScript. My ultimate goal is to is to create decentralized browser extensions (like MetaMask) for the Steem, EOS, and Tron blockchain. However, instead of making a wallet or a block explorer, I want to make simple games. I've also talked about making a powerful filter for custom Steem trending tabs and a decentralized exchange. Those are still on the table (although a bit more boring).
I tried to do this a while back but got completely overwhelmed. I was trying to learn many new technologies all at the same time: JavaScript, Node.js, Steem API, Chrome-Extensions, and HTML. Let me tell you, it was a flippin disaster. Learning one of these is complicated enough. Trying to learn them all at the same time and connect them together is near impossible. Therefore, I've taken a step back and have been learning just JavaScript and HTML. So far I'm enjoying my time. JavaScript is a nice little language.
Odd-Point Combat
I started with the project that is by far the most simple: basically just rock, paper, scissors with a twist. In my limited experience dealing with Chrome Extensions I learned that one must separate all JavaScript from the HTML file, and put that code into it's own JS file. For example:
Block, Paper, Scissors
???
???
???
???
Alright well I'm officially annoyed as fuck. I just spent like 30 minutes trying to figure out how to post that code. You have to escape the HTML so that it doesn't try to load on the page. Apparently, escaping HTML is no easy feat.
Is supposed to work but doesn't.
Is also supposed to work but doesn't.
Is also supposed to work, but doesn't, and it's deprecated.
In the end I had to put 4 spaces in front of every line by hand to get it to show. If you know how let me know... just kidding I realized I can just add a tab to my code in Atom, because my tabs are equivalent to spaces. I R SMRT.
ANYWAY!
The above code is all of the HTML of my little learning project. As you can see the only script tag I am allowed to use simply points to the file that contains the JavaScript logic.
I'll post that here (script.js):
var reverse = false; var HIT_POINTS = 1000; var SOFT_DAMAGE = 100; var HARD_DAMAGE = 200; var softCounter = SOFT_DAMAGE; var hardCounter = HARD_DAMAGE;
var player1 = { name:"edicted", hp:HIT_POINTS, choice:"" };
var player2 = { name:"shadow", hp:HIT_POINTS, choice:"" };
function menu(){ var option = document.getElementById('menu').value; if(option == "mage"){ reverse = false; document.getElementById("attack1").value = "fire"; document.getElementById("attack1_label").innerHTML = "Fire Ball!"; document.getElementById("attack2").value = "lightning"; document.getElementById("attack2_label").innerHTML = "Lightning Bolt!"; document.getElementById("attack3").value = "water"; document.getElementById("attack3_label").innerHTML = "Water Blast!"; document.getElementById("attack4").value = "wind"; document.getElementById("attack4_label").innerHTML = "Wind Gust!"; document.getElementById("attack5").value = "earth"; document.getElementById("attack5_label").innerHTML = "Earth Stomp!"; } else if(option == "melee"){ reverse = false; document.getElementById("attack1").value = "charge"; document.getElementById("attack1_label").innerHTML = "Charge!"; document.getElementById("attack2").value = "lunge"; document.getElementById("attack2_label").innerHTML = "Lunge!"; document.getElementById("attack3").value = "slash"; document.getElementById("attack3_label").innerHTML = "Slash!"; document.getElementById("attack4").value = "riposte"; document.getElementById("attack4_label").innerHTML = "Riposte!"; document.getElementById("attack5").value = "deflect"; document.getElementById("attack5_label").innerHTML = "Deflect!"; } else if(option == "yolo"){ reverse = true; document.getElementById("attack1").value = "jeep"; document.getElementById("attack1_label").innerHTML = "Jeep!"; document.getElementById("attack2").value = "helo"; document.getElementById("attack2_label").innerHTML = "Helo!"; document.getElementById("attack3").value = "bomber"; document.getElementById("attack3_label").innerHTML = "Bomber!"; document.getElementById("attack4").value = "fighter"; document.getElementById("attack4_label").innerHTML = "Fighter!"; document.getElementById("attack5").value = "tank"; document.getElementById("attack5_label").innerHTML = "Tank!"; } else if(option == "spock"){ reverse = false; document.getElementById("attack1").value = "rock"; document.getElementById("attack1_label").innerHTML = "Rock!"; document.getElementById("attack2").value = "lizard"; document.getElementById("attack2_label").innerHTML = "Lizard!"; document.getElementById("attack3").value = "paper"; document.getElementById("attack3_label").innerHTML = "Paper!"; document.getElementById("attack4").value = "scissors"; document.getElementById("attack4_label").innerHTML = "Scissors!"; document.getElementById("attack5").value = "spock"; document.getElementById("attack5_label").innerHTML = "Spock!"; } else if(option == "whale"){ reverse = false; document.getElementById("attack1").value = "whale"; document.getElementById("attack1_label").innerHTML = "Whale!"; document.getElementById("attack2").value = "plankton"; document.getElementById("attack2_label").innerHTML = "Plankton!"; document.getElementById("attack3").value = "orca"; document.getElementById("attack3_label").innerHTML = "Orca!"; document.getElementById("attack4").value = "minnow"; document.getElementById("attack4_label").innerHTML = "Minnows!"; document.getElementById("attack5").value = "dolphin"; document.getElementById("attack5_label").innerHTML = "Dolphins!"; } if(reverse){ softCounter = HARD_DAMAGE; hardCounter = SOFT_DAMAGE; } else { softCounter = SOFT_DAMAGE; hardCounter = HARD_DAMAGE; } }
function attack(){ if(player1.choice == ""){ if (document.getElementById('attack1').checked) player1.choice = document.getElementById('attack1').value; else if (document.getElementById('attack2').checked) player1.choice = document.getElementById('attack2').value; else if (document.getElementById('attack3').checked) player1.choice = document.getElementById('attack3').value; else if (document.getElementById('attack4').checked) player1.choice = document.getElementById('attack4').value; else if (document.getElementById('attack5').checked) player1.choice = document.getElementById('attack5').value; else alert("No attack selected!"); } else if (player2.choice == ""){ var attack = document.querySelector('input[name = "attack"]:checked'); player2.choice = attack.value; } else alert("Both players have chosen!"); update(); }
function resolve(){ /* attack1 counters attack2 and attack4 attack2 counters attack5 and attack3 attack3 counters attack1 and attack5 attack4 counters attack3 and attack2 attack5 counters attack4 and attack1 //*/ if(player1.choice != "" && player2.choice != ""){ if(player1.choice == document.getElementById('attack1').value){ if(player2.choice == document.getElementById('attack2').value) player2.hp -= softCounter; if(player2.choice == document.getElementById('attack4').value) player2.hp -= hardCounter; if(player2.choice == document.getElementById('attack3').value) player1.hp -= softCounter; if(player2.choice == document.getElementById('attack5').value) player1.hp -= hardCounter; } if(player1.choice == document.getElementById('attack2').value){ if(player2.choice == document.getElementById('attack5').value) player2.hp -= softCounter; if(player2.choice == document.getElementById('attack3').value) player2.hp -= hardCounter; if(player2.choice == document.getElementById('attack1').value) player1.hp -= softCounter; if(player2.choice == document.getElementById('attack4').value) player1.hp -= hardCounter; } if(player1.choice == document.getElementById('attack3').value){ if(player2.choice == document.getElementById('attack1').value) player2.hp -= softCounter; if(player2.choice == document.getElementById('attack5').value) player2.hp -= hardCounter; if(player2.choice == document.getElementById('attack4').value) player1.hp -= softCounter; if(player2.choice == document.getElementById('attack2').value) player1.hp -= hardCounter; } if(player1.choice == document.getElementById('attack4').value){ if(player2.choice == document.getElementById('attack3').value) player2.hp -= softCounter; if(player2.choice == document.getElementById('attack2').value) player2.hp -= hardCounter; if(player2.choice == document.getElementById('attack5').value) player1.hp -= softCounter; if(player2.choice == document.getElementById('attack1').value) player1.hp -= hardCounter; } if(player1.choice == document.getElementById('attack5').value){ if(player2.choice == document.getElementById('attack4').value) player2.hp -= softCounter; if(player2.choice == document.getElementById('attack1').value) player2.hp -= hardCounter; if(player2.choice == document.getElementById('attack2').value) player1.hp -= softCounter; if(player2.choice == document.getElementById('attack3').value) player1.hp -= hardCounter; } player1.choice = ""; player2.choice = ""; update(); if( player1.hp <= 0 ){ gameOver(player1); } if( player2.hp <= 0 ){ gameOver(player2); } } else { alert("Both players need to pick.") } }
function gameOver(deadPlayer){ alert("GAME OVER! " + deadPlayer.name + " has been vaporized!"); }
function update(){ document.getElementById("p1").innerHTML = player1.name + " has " + player1.hp + " hp "; document.getElementById("p2").innerHTML = player2.name + " has " + player2.hp + " hp "; document.getElementById("p3").innerHTML = player1.name + " : " + player1.choice; document.getElementById("p4").innerHTML = player2.name + " : " + player2.choice; }
document.getElementById("attackButton").onclick = attack; document.getElementById("resolveButton").onclick = resolve; document.getElementById("menu").onchange = menu;
update();
Now what?
If you're so inclined, copy and paste the HTML file into a text editor like Notepad and save the file as "combat.html". Then copy paste the JavaScript into Notepad and save it as "script.js" in the same folder. You should now be able to double click the combat.html file and this "website" should pop up in your default browser:
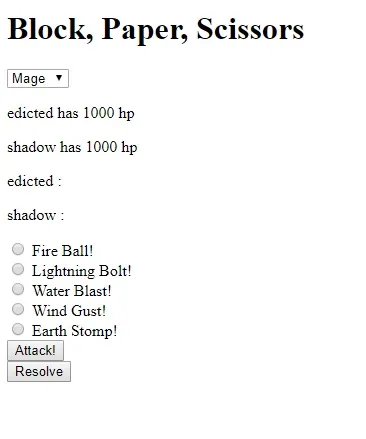
This is basically a rock, paper, scissors game with five options instead of three. You can change the names of the attacks by clicking the dropdown menu. Instead of each ability equally countering two other abilities, I've programmed a "hardCounter" and a "softCounter". Both players start at 1000 life. a softCounter does 100 damage and a hardCounter does 200 damage. Whichever player reaches 0 hp first loses.
Proof-of-Brain
This game is too simple to implement proof-of-brain at the user level. However, maybe "simple" is a bad word to use because Steem Monsters is pretty complicated and it still doesn't utilize proof-of-brain. Regardless, if I happened to find other programmers to help me with my games they could start working on a particular "fork". Say someone wanted to flush out the mage version. Instead of radio buttons they would add pictures of the abilities. Then, perhaps later, they go even further and add graphics to the game. What I'm trying to do here is create the seeds of proof-of-brain that the Steem community can build on and get paid for their hard work.
So What?
Yes, this "game" is garbage. It's a one player rock, paper, scissors vs yourself. However, once I connect it to Steem I will make it two player, and I will allow bets to be placed on the winner. With the same logic I use to implement this crappy little game, I will make a myriad of decentralized apps on the blockchain. This is the power of the Steem blockchain and module object oriented programming. Stay tuned.
Return from Block, Paper, Scissors to edicted's Web3 Blog